1
/
of
1
MPLTOTO
MPLTOTO: Situs Toto Togel & Slot Gacor Resmi
MPLTOTO: Situs Toto Togel & Slot Gacor Resmi
Regular price
Rp 10.000.000,00
Regular price
Rp 50.000.000,00
Sale price
Rp 10.000.000,00
Unit price
/
per
Couldn't load pickup availability
MPLTOTO merupakan situs toto togel online, slot online gacor resmi terpercaya yang menyediakan pasaran togel online terlengkap dan provider slot online terbaik dengan winrate tertinggi.
Share
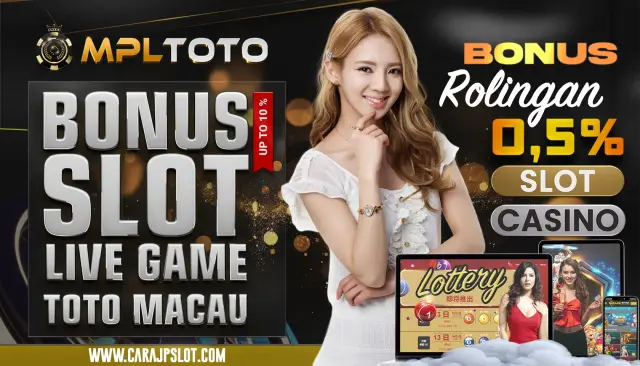